The Visual Studio Code editor, Python interpreter and built-in debugging tool
Thus far all the exercises on this course have been completed directly on the course pages in embedded editor windows. Programming in the browser is very suitable for the very first steps in programming, but now it is time to start using a separate editor especially made for programming.
There are dozens of different editors that are suited to programming. On this course we will use the Visual Studio Code editor, which has been gaining traction in recent years.
Please install the Visual Studio Code editor on your own computer now. You may also need to install Python and the Visual Studio Code plugin for Python. You will also need the TMC plugin, which will take care of running the tests that go with the exercises. In the TMC plugin, select MOOC as the organization and Python Programming 2021 as the course.
Here is a guide to installing and running all of these. Read the instructions on working on and submitting exercises, and then complete the task below:
Executing code
In Visual Studio Code, the easiest way to execute your code is clicking on the triangle in the upper right hand corner. Sometimes it happens that some program is left running in the background, maybe waiting for input from the user or stuck in an infinite loop, without you realising it. You might only notice this when trying to execute the next program, which simply will not run because the previous execution is still hogging up resources. A quick fix is pressing on the keys Control+C at the same time, which stops the execution of any ongoing process. The next program should now run fine.
The interactive Python interpreter
One of the most important tools for any Python programmer is the interactive Python interpreter.
Starting up the interpreter may depend on your platform. In a Linux or Mac system, you can type in python3
in the terminal. In Windows, the command on the command line might be python
. Firing up the interpreter on a Mac looks like this:

It is also possible to start the interpreter inside Visual Studio Code. First you should execute a program by clicking on the triangle. This should open a Terminal section on your screen, where you can now type in python3
(or python
):
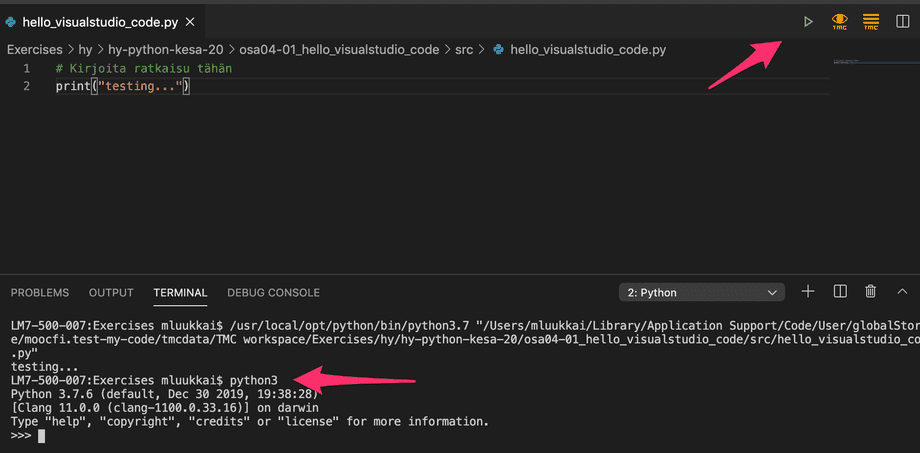
You can also try a browser-based Python interpreter, such as https://www.python.org/shell/.
The interpreter is a way of executing Python code line by line as soon as you write it. When you write a line of code and press Enter, the interpreter executes the code right away and shows you the results:

Any Python code that can be written in a file can also be written in the interpreter. You can even assign variables and define methods:
>>> t = [1,2,3,4,5]
>>> for number in t:
... print(number)
...
1
2
3
4
5
>>> def absolute_value(number):
... if number<0:
... number = -number
... return number
...
>>> x = 10
>>> y = -7
>>> absolute_value(number)
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
NameError: name 'number' is not defined
>>> absolute_value(x)
10
>>> absolute_value(y)
7
>>>
The interpreter is best suited to making small checks. For instance, you can try out functions and methods, or check if they exist at all:
>>> "TextYteXt".toupper()
Traceback (most recent call last):
File "<stdin>", line 1, in <module>
AttributeError: 'str' object has no attribute 'toupper'
>>> "TextYteXt".upper()
'TEXTYTEXT'
>>>
If there is a method you need, and you can almost remember its name, it's sometimes faster to skip Google and use the dir
function in the interpreter. It tells you which methods are available to use on a given object:
>>> dir("this is a string")
['__add__', '__class__', '__contains__', '__delattr__', '__dir__', '__doc__', '__eq__',
'__format__', '__ge__', '__getattribute__', '__getitem__', '__getnewargs__', '__gt__',
'__hash__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__', '__lt__',
'__mod__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__',
'__rmod__', '__rmul__', '__setattr__', '__sizeof__', '__str__', '__subclasshook__',
'capitalize', 'casefold', 'center', 'count', 'encode', 'endswith', 'expandtabs', 'find',
'format', 'format_map', 'index', 'isalnum', 'isalpha', 'isascii', 'isdecimal', 'isdigit',
'isidentifier', 'islower', 'isnumeric', 'isprintable', 'isspace', 'istitle', 'isupper', 'join',
'ljust', 'lower', 'lstrip', 'maketrans', 'partition', 'replace', 'rfind', 'rindex', 'rjust','rpartition', 'rsplit', 'rstrip', 'split', 'splitlines', 'startswith', 'strip', 'swapcase',
'title', 'translate', 'upper', 'zfill']
Python strings have a lot of methods available, as you can see above. At this point it might be best to ignore any methods that have underscores in their names, but the rest may well prove useful. Some of them you can figure out by trying them out, and the rest you can search for online.
Python lists don't have quite so many methods:
>>> dir([])
['__add__', '__class__', '__contains__', '__delattr__', '__delitem__', '__dir__', '__doc__',
'__eq__', '__format__', '__ge__', '__getattribute__', '__getitem__', '__gt__', '__hash__',
'__iadd__', '__imul__', '__init__', '__init_subclass__', '__iter__', '__le__', '__len__',
'__lt__', '__mul__', '__ne__', '__new__', '__reduce__', '__reduce_ex__', '__repr__',
'__reversed__', '__rmul__', '__setattr__', '__setitem__', '__sizeof__', '__str__',
'__subclasshook__', 'append', 'clear', 'copy', 'count', 'extend', 'index', 'insert', 'pop',
'remove', 'reverse', 'sort']
>>>
Let's try a few of them, reverse
and clear
seem promising:
>>> numbers = [1,2,3,4,5]
>>> numbers.reverse()
>>> numbers
[5, 4, 3, 2, 1]
>>> numbers.clear()
>>> numbers
[]
As you can see, these methods do pretty much what you would expect based on their names.
Notice how the interpreter doesn't print out anything when you run the command numbers.reverse()
. This is because the interpreter prints something out only if the line of code has a value. The reverse()
method here doesn't return any value.
In the above example we printed out the value of the list numbers
by typing in just the name of the variable. In fact, it is rarely necessary to explicitly type in print
commands in the interpreter. You can include them if you want to, however.
Remember to close the interpreter when you are finished. The commands quit()
or exit()
will close it, as will the key combo Control+D (Linux/Mac) or Control+Z (Windows). Especially in Visual Studio Code this is important, as trying to execute another Python program while the interpreter is still running results in a rather cryptic error message:
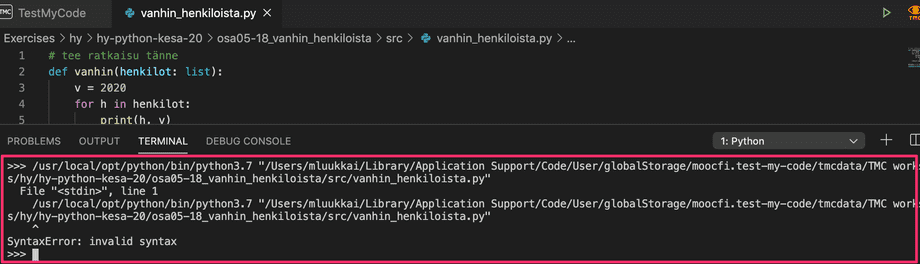
The built-in debugger
We have already spent a fair amount of time and effort on developing debugging skills, mainly through print statement debugging. The Visual Studio Code editor has another tool at your disposal: a built-in visual debugger.
To start debugging, first you have to define a breakpoint in your code. A breakpoint is a point in your program where the debugger halts execution. You can set a breakpoint by clicking on the left edge of any line in your program.
The following example is a slightly faulty attempt at solving the exercise The sum of consecutive numbers in the previous part. There is a breakpoint on line 5:
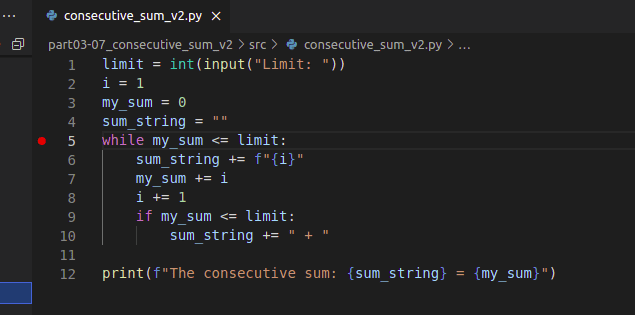
After the breakpoint has been set, choose Start debugging from the Run menu. This opens a list of options, where you should select Python File:
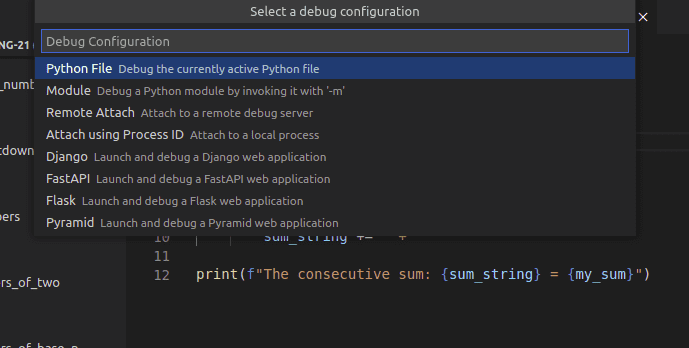
This starts the debugger, which executes your code as usual until the execution reaches a breakpoint and halts. If your code asks for input, remember to type that in in the terminal:
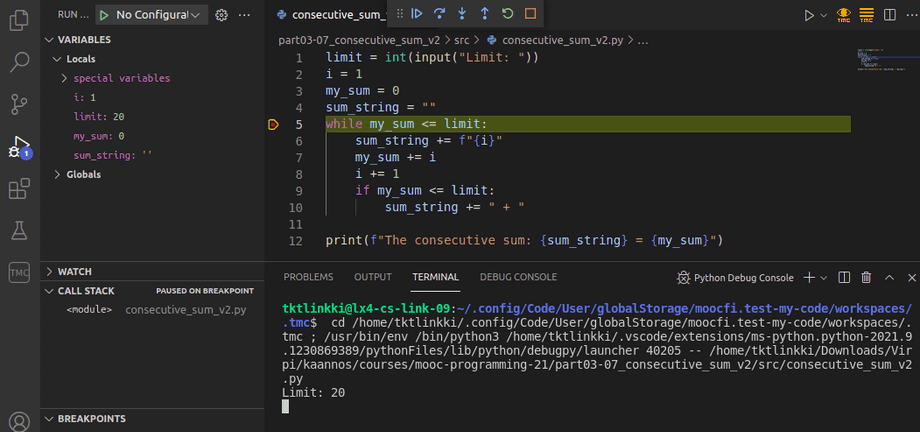
On the left there is now the Variables view, which contains the current values of all variables active in the code. You can proceed with the execution line by line by clicking on the down arrow, which is labelled Step into.
In the image below the loop in the code has already been repeated a few times:
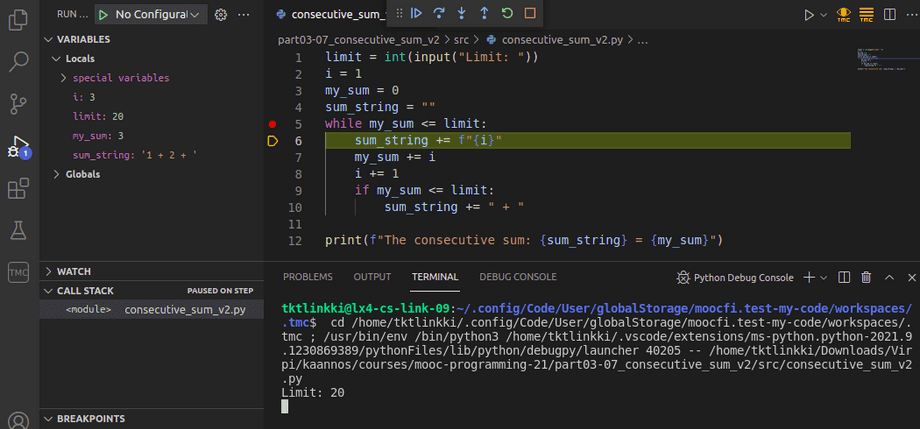
The debugger has a Debug console tab, which lets you evaluate expressions with the current values stored in the variables. For example, you can check the value of the Boolean expression in the condition of the loop:
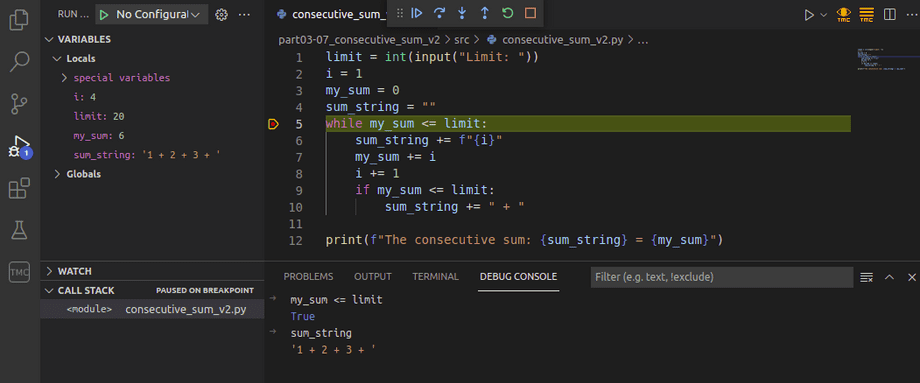
You can include several breakpoints in your program code. When execution has halted, you can start it again by clicking on the blue triangle. Execution proceeds until it reaches the next breakpoint.
The built-in visual debugger is a good alternative to debugging print statements. It is up to you which you choose to employ more in the future. Each programmer has their own preferences, but it's always a good idea to try different options before settling on any single solution.
You can check your current points from the blue blob in the bottom-right corner of the page.